Is Python Requests blocking?
Python is an open-source programming language that has a wide range of libraries and modules that help developers build applications faster and more efficiently. One such library is Python Requests, which is used to send HTTP requests to web servers and retrieve data.
When it comes to sending HTTP requests, there are two types of approaches: blocking and non-blocking. In a blocking approach, the program waits for a response from the server before moving on to the next line of code. On the other hand, in a non-blocking approach, the program continues to run while waiting for a response from the server.
Python Requests, by default, uses a blocking approach. This means that when you send a request using the Requests library, the program will wait for a response from the server before moving on to the next line of code.
import requests
response = requests.get('https://www.example.com')
print(response.content)
In the above code snippet, the program will wait for a response from 'https://www.example.com' before moving on to the next line of code, which is printing the content of the response. This is because Requests uses a blocking approach by default.
However, there are ways to make Requests non-blocking. One such way is by using the gevent library. Gevent is a concurrency library for Python that provides a way to run multiple greenlets (lightweight threads) at the same time.
import gevent
import requests
urls = [
'https://www.example.com',
'https://www.google.com',
'https://www.github.com'
]
def print_content(url):
response = requests.get(url)
print(response.content)
jobs = [gevent.spawn(print_content, url) for url in urls]
gevent.joinall(jobs)
In the above code snippet, we are using gevent to run multiple HTTP requests at the same time. We define a function called print_content that sends an HTTP request and prints the content of the response. We then create multiple greenlets using gevent.spawn and pass in the print_content function along with the URLs we want to request. Finally, we use gevent.joinall to wait for all the greenlets to finish.
So, to sum up, Python Requests uses a blocking approach by default, but it is possible to make it non-blocking by using a concurrency library like gevent.
Want to learn more? Check this out!
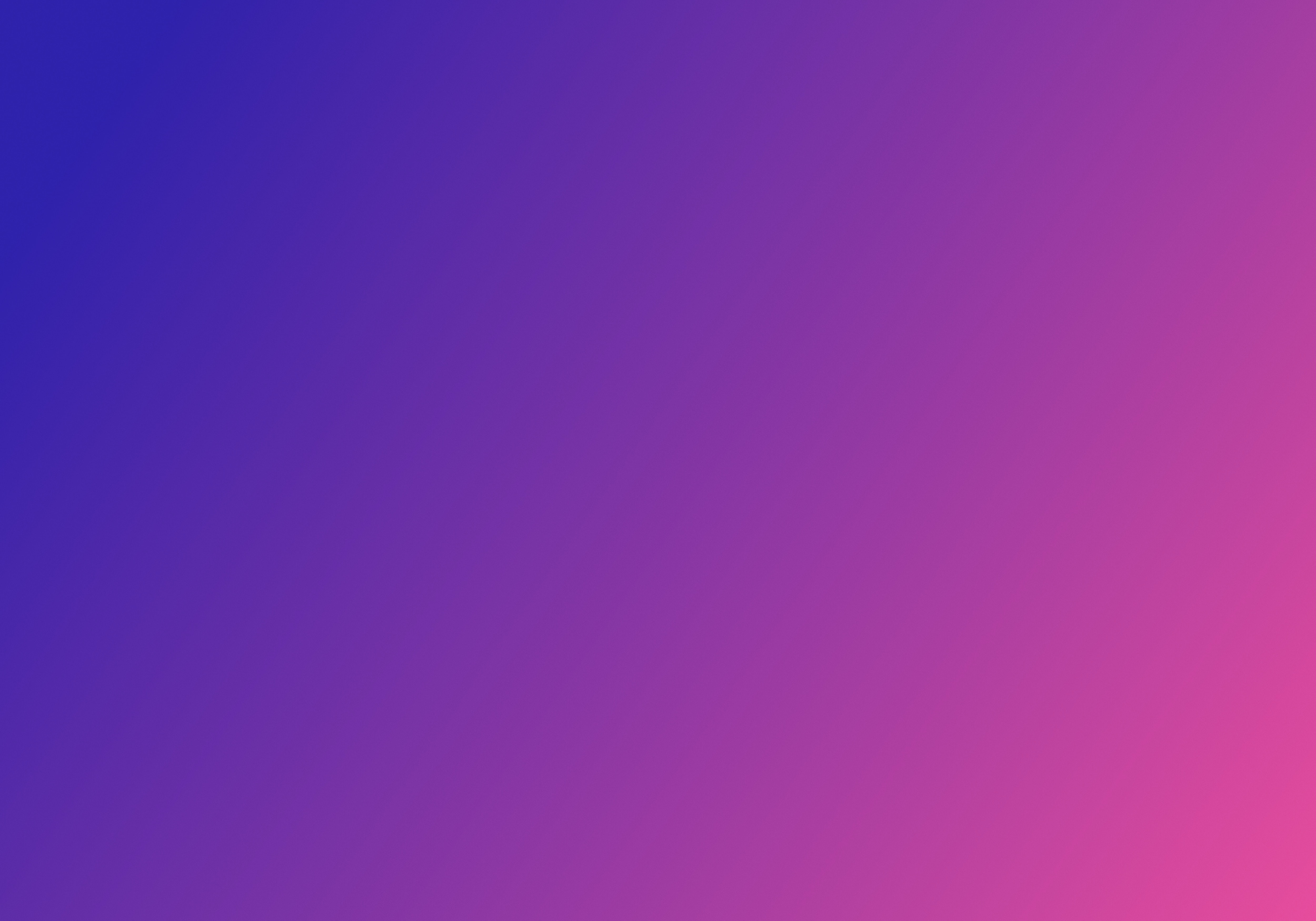